Thanks for the replies. I think my original post was a bit unclear, the problem is quite subtle and it isn't a problem with intersect generally but rather:
-
Explode doesn't always merge geometry properly. In the image below an explosion will fail to cut the top face in the left model. The grouped edges in the right model is a subset, and in this case an explosion will split the top face into four faces as we expect. So, for explosion to be robust the geometry needs to be as simple as possible.
-
Intersect_with
doesn't provide all edges for coplanar cuts.
The original problem was a combination of 1 and 2.
If the two groups are cut from the UI, the resulting edges represents all coplanar cuts. So, what's the difference between the UI intersect and the API intersect? It turns out that the resultant edges from UI intersect is (conceptually):
intersect_with g0, g1 + intersect_with g1, g0
Thus, the solution is to make two calls to intersect_with
in the API. The script below properly cuts the two pipes.
` def mergeGroups(ent, g0, g1)
ng = ent.add_group
ng.entities.add_instance(g0.entities.parent, g0.transformation)
ng.entities.add_instance(g1.entities.parent, g1.transformation)
g0.erase!
g1.erase!
nt1 = ng.entities[0]
nt2 = ng.entities[1]
nt1.explode
nt2.explode
return ng
end
def intersect(ent, g0, g1)
eg = ent.add_group
g0.entities.intersect_with false, g0.transformation, eg , g1.transformation , true, g1
return eg
end
def cutGroupPair(ent, g0, g1)
ge0 = intersect(ent, g0, g1)
ge0.transform! g1.transformation
ge1 = intersect(ent, g1, g0)
ge1.transform! g0.transformation
g00 = mergeGroups(ent, ge0, g0)
g11 = mergeGroups(ent, ge1, g1)
ng = mergeGroups(ent, g11, g00)
return ng
end
mod = Sketchup.active_model
ent = mod.entities
sel = mod.selection
cutGroupPair(ent, sel[0], sel[1])`
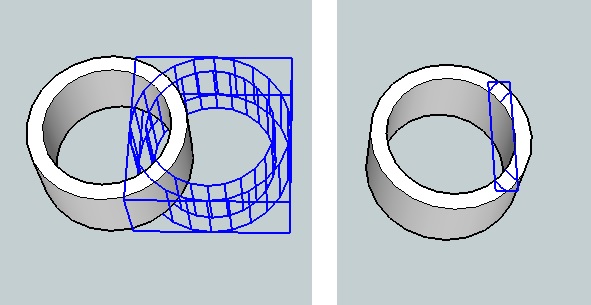