Yes, ruby does have a modulo operator - I said Dynamic Components doesn't provide a modulus function.
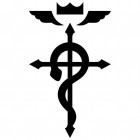
Posts made by danielbowring
-
RE: Hide/Show only every Nth Copied Instance?
-
RE: Hide/Show only every Nth Copied Instance?
You should be using a modulo operation for this. A modulo operation will give the "remainder" of integer division. SketchUp doesn't provide a mod function for DCs, so you'll have to create it as such:
a - (n * FLOOR(a/n))
where a is the index and you want to hide every N.
For example, if you have
HIDDEN=COPY - (3 * FLOOR(COPY/3))==0
, you'll get:
HIDDEN, VISIBLE, VISIBLE, HIDDEN
.
You can change the compared value (in the above example, 0) to be the "offset", but it must be less than n.
For example, if you have
HIDDEN=COPY - (3 * FLOOR(COPY/3))==2
, you'll get:
VISIBLE, VISIBLE, HIDDEN, VISIBLE, ...
.
-
RE: Guidelines for adding a method to existing classes?
@tt_su said:
Note that extending
Entity
instances would not be allowed by EW because the instances are global to everyone using the SketchUp environment. But for other types such as Point3d, Vector3d, String etc that would work.Here
delegators
can be useful.require 'delegate' module DanielB class SampleFace < DelegateClass(Sketchup;;Face) def inner_loops return loops.find_all { |l| l != outer_loop } end end def self.demo face = Sketchup.active_model.selection.find { |e| e.is_a?(Sketchup;;Face) } return if face.nil? face = SampleFace.new(face) puts face.inner_loops end end
-
RE: Need Help: DC constrain min/max scaling size
If you want to avoid if statements, you can use
SMALLEST
andLARGEST
like so=SMALLEST(MAX_VALUE, LARGEST(MIN_VALUE, value))
-
RE: Migrating plugins to SketchUp 2013
Epic bump, but is there any news on this? Was upgrading the installation ruby put on the backburner? Should we still be expecting this sometime in the future (or a complete library at least)?
To me at least, this seems like a really important feature - plugins and the community around them are a powerful part of sketchup.
-
RE: [Project] Community Documentation Effort
@tt_su said:
Just so you're aware, we're looking into new solution for the API docs. I don't know of any time frame, yet though.
That's great! I'll continue working on this in the meantime, though. There's still a lot of community info to round-up and it may even come of use once you guys have whatever it is you're planning sorted.
Here's hoping you deprecate this soon
-
RE: [Project] Community Documentation Effort
@rich o brien said:
Is there a means of embedding that on a webpage here?
I doubt there will be any particular mechanism (as there is for gists) because of demand reasons.
-
RE: [Project] Community Documentation Effort
Only other one I'm aware of is JimFoltz's, but his doesn't allow the kind of stuff a wiki can.
-
[Project] Community Documentation Effort
Hey,
It's no secret that the documentation for the ruby API has its problems. We've asked for problems to be fixed (and we had comments for a while!) but it seems the dev team is too busy with other things to maintain it (which, in-of-itself is a good thing - SketchUp improvements!)
So, I've started working on a community written API documentation. I've mostly decided on a style and layout, but before things are set in stone I'd like to have some input from other people.
The project is hosted on Github and is made of of two parts:
- A Wiki to hold information on all the classes, modules, methods, ... as well as tutorials and guides
- A repository for non-trivial examples and resources (such as images)
I'll be waiting a few days before I start the real effort so I can (hopefully) get some feedback from everyone. I suggest looking at the wiki page for
UI
,UI::WebDialog
andUI::WebDialog#write_image
for an example of each main "type" of page (module, class, method).I know a lot of the information is scattered around already (especially here on these forums), but hopefully we can consolidate it into one, organised location.
-
RE: How to Use Graphing Gem in SketchUp Plugin
@tt_su said:
whut whut?? A bug you say?
Ah! Looks like it is a bug, but only in the documentation. The actual argument order is:
- filepath
- quality (0-100, 100 being best quality)
- top_left_x
- top_left_y
- bottom_right_x
- bottom_right_y
It previously appeared to be poor quality because I was using the origin (0,0) which gave the worst quality, and it gave the wrong size because when the size is invalid (or missing) it just gives the full client area.
PNG's always came out great because they are a lossless format.Props to Aerilius for guessing right
-
RE: How to Use Graphing Gem in SketchUp Plugin
@aerilius said:
There is no quality or compression argument (?). Or did someone try
:compression
from view.write_image, maybe it's undocumented?Wrong
write_image
- I'm talking aboutdialog.write_image
Edit: Wait, I'm the one misunderstanding!
Yea, as far as I know there is no compression argument for it.Edit2: It doesn't appear to take a hash-options.
However, the poorer quality only seems to happen for JPEGs. Saving it as a PNG maintains the quality but completely ignores the size arguments. -
RE: How to Use Graphing Gem in SketchUp Plugin
If you're feeling especially adventurous, you could try using a
canvas
tag within a web dialog (explorercanvas is your friend) and then useWebDialog#write_image
.Edit: Wow, I actually just had a play with that and the image quality is horrible. Is that just how it is?
Edit2: Not passing any arguments other than file location fixes the quality, but means you have to capture the full client area (including scrollbars, if they are present) -
RE: Change plugin variables within Undo
you could store the value/flag on the model instead, then it would also change with the undo steps
model = Sketchup.active_model model.set_attribute('maricanis', 'dl_materials_changed', true) #... model.get_attribute('maricanis', 'dl_materials_changed', false)
-
RE: Connecting Sketchup's ruby with MYSQL database
Oh, if you're only intending to use the data locally, then any remote data source would be overkill. If you want to just save it to the model, you can just save it as an attribute to the model.
Entity#(g|s)et_attribute
can store a few different types, includingPoint3d
objects.model = Sketchup.active_model # Save array of points to the model model.set_attribute('my-points', 'some-key', [ORIGIN, Geom;;Point3d.new(1, 2, 3)]) # Get the points from the model, or an empty list if none are present my_points = model.get_attribute('my-points', 'some-key', [])
-
RE: Connecting Sketchup's ruby with MYSQL database
Off topic but tangent: Setting that up is going to be hell (version support, requirements, ...), and it's going about it the "wrong" way anyway. You should look at making a web service and query information using that, not using sql directly. This will be easier in the long term and easier to manage auth/security. What's more, you'll be able to deal with the information using
net/http
and then whatever data serialization method you use.If you're really determined to get a direct connection to work, you're missing the header files for ODBC. You'll need to go get a distro/version compatible that's with your compiler and sketchups ruby. There's a version by Microsoft available somewhere and I'd guess that it would be a good choice, but I've never researched/tried.
I do recommend the web-service approach, though.
-
RE: RBZ and Components library
@driven said:
for a mac you can create a symbolic link the will show the skps in the 'Components Browser'
lnk_skps = %x(ln -s "#{Sketchup.find_support_file("Plugins") << "/your_folder/your_skps"}" "#{Sketchup.find_support_file('Components')}" 2>&1)
is there not a windows equivalent?
johnFor Vista and higher:
mklink /D LinkName TargetPath
XP "supports" a similar feature called junction points, but I don't think it's available by default.
For XP:
links LinkName TargetPath
For Vista and higher:
mklink \J LinkName TargetPath
-
RE: Aborting a .rbz install
@driven said:
I'm under the impression Windows will see it as a space and error out
Error: #<NoMethodError: undefined method
windows' for #Object:0x56b89ec>`Depends what encoding you save it in.
Western (Windows 1252) gives this:
Error; #<SyntaxError; (eval);5231;in `load'; path/to/nbsp.rb;1; Invalid char `\240' in expression>
UTF-8, however, will load and work fine
windows use = "o.~" puts "Success #{windows use.inspect}" # -> load 'path/to/nbsp.rb' Success "o.~" true
Note: Browsers will replace the character in question (160, "non-breaking space") with a regular space (32, "space"), but I did correct for this for this test.
-
RE: [Question] DC Size and New Definitions
Possibly related: This animation behavior.
Click any except the first to see they animate individually, but when the first is clicked it will animate all copies.
-
RE: Aborting a .rbz install
@driven said:
having 'only works on mac' should be enough, but never is.
I'm just wanting to avoid the 'this isn't working' posts from 'PC' users.
Beside anything else, it would be trivial for SU to pre-screen for files marked as os dependant.
john
Note the
if is_mac?
at the end ofPluginModule
. That will prevent the plugin from "loading" anything more. Same if you just don't register theSketchupExtension
. You could also write a setting to prevent it re-showing the message usingSketchup.write_default
/Sketchup.read_default
Edit: Demo
module MyModule puts "This section will never be executed!" end if false # because of this! puts "But this will!" puts defined?(MyModule)
Gives
But this will! nil